Dynamic Interface Mesh (DIM)
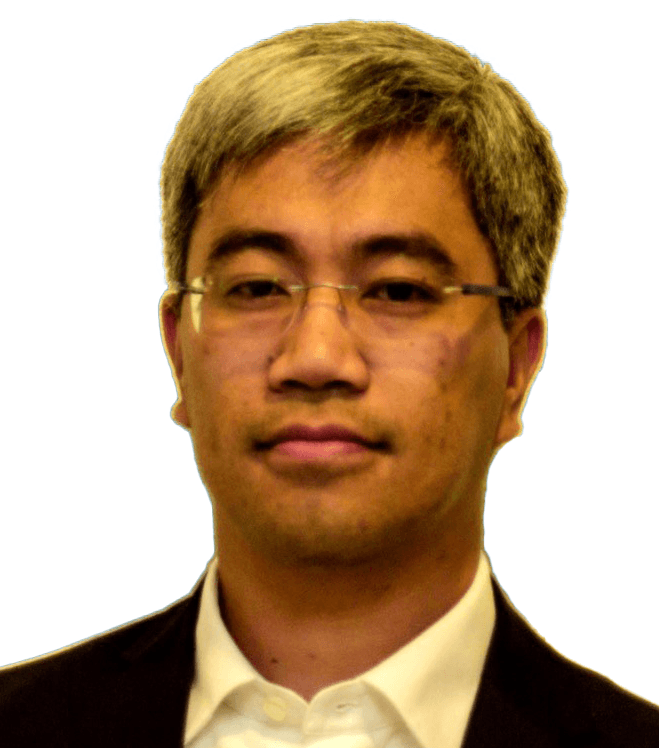
- Published on
Introduction
As modern frontend systems scale across teams, platforms, and surfaces, the need for flexible, context-aware UI composition becomes increasingly urgent. The Dynamic Interface Mesh (DIM) addresses this challenge by enabling the runtime composition of user interfaces—allowing fragments, components, and views to be assembled, adapted, and orchestrated dynamically.
DIM decouples rendering logic from deployment logic. It makes the frontend more like a data-driven mesh of independently executable and pluggable views that can be served by different teams, loaded on demand, and even resolved conditionally based on runtime capabilities.
Where CEL centralizes business logic, DIM focuses on UI layout, hierarchy, and component-level federation. It treats views as units of composition that can be resolved at runtime, based on context, capability, or configuration.
Why We Need DIM
UI complexity increases as frontends stretch across mobile, desktop, kiosk, embedded surfaces, and internationalized markets. Traditional component composition (e.g., static imports or monolithic layout trees) doesn't scale across these boundaries. DIM provides solutions to many of these scaling and flexibility problems:
On-demand UI resolution: Views are dynamically loaded at runtime based on contextual factors like route, device type, user permissions, or feature flags. This minimizes the initial payload, reduces render blocking, and allows interfaces to adapt intelligently to user needs.
Composition as configuration: Instead of hardcoding view hierarchies, interfaces can be assembled from JSON or remote schemas. This makes UI structure more portable and adjustable without redeploying entire apps.
Team-level UI ownership: DIM empowers cross-functional teams to own view fragments independently. Each team can deploy UI modules without needing coordination from a monolithic frontend team, improving velocity and autonomy.
Better separation of layout vs behavior: By abstracting layout logic away from CEL-based behavior modules, developers can independently evolve structure and logic. Designers and engineers can focus on visual hierarchy without being blocked by business logic integration.
Historical Context and Prior Art
Static Composition in Monoliths
Early React apps, Angular SPAs, and server-rendered UIs relied on static component trees wired together via imports. While straightforward, this design doesn't scale when views must be swapped, injected, or federated.
Micro Frontends
Micro frontends attempted to address UI modularity by letting teams deploy entire apps per domain (e.g. account, orders, settings). But they came with heavy runtime and integration costs, and often lacked fine-grained UI composition.
Web Component Registries and Runtime UIs
Tools like single-spa, OpenComponents, and even custom module loaders explored runtime UI registration. DIM learns from these attempts by integrating a runtime mesh with fine-grained scope, lifecycle isolation, and dependency resolution.
Component Federation via Module Federation
Webpack Module Federation introduced a powerful way to share components across builds. DIM builds on this by adding dynamic layout resolution, runtime wiring, and coordination with CEL and ECN.
Architecture Overview
DIM operates as a runtime composition system where each view fragment is treated as a mesh node that can be resolved, configured, and rendered independently. The system decouples layout from logic, and runtime view resolution from build-time bundling. This is especially critical for large-scale applications where UI elements must change dynamically without full redeployment.
DIM is built around the concept of a Mesh Resolver, which is responsible for determining what content gets rendered in which region of the UI shell. It supports:
- Lazy loading of views based on route or state
- Policy-driven rendering (e.g., user role or device)
- Dynamic substitution of content (e.g., based on feature flags or A/B experiments)
Here’s how the architecture maps to implementation and runtime behavior:
+------------------------------+
| Route Handler |
+------------------------------+
↓
[DIM Mesh Resolver]
↓
+------------------------------+
| View Shell (App Shell) |
| ┌────────┬────────┬───────┐ |
| │ Header │ Body │ Footer│ |
| └────────┴────────┴───────┘ |
+------------------------------+
↓
[Resolved Mesh Nodes]
↙ ↘
[Component A] [Component B]
How Code Examples Map to the Architecture
Architecture Layer | Code Example | Explanation |
---|---|---|
Route Handler | Implicit in navigation system | Triggers the entire DIM resolution process, often via a router or lifecycle hook. |
DIM Mesh Resolver | resolveMesh('CheckoutSummary') | Dynamically resolves the registered component based on configuration and runtime context. |
Component Registry | registerMeshComponent(...) | Registers components to the mesh so they can be discovered at runtime. |
View Shell / Layout | <MeshRegion name="main" /> | Declares a target zone in the layout where dynamic content will be injected. |
Policy Evaluation | policy={regionPolicy} or policy="admin-only" | Determines visibility or access based on user roles, features, or runtime conditions. |
Fallback Handling | fallback={<Loading />} or <ErrorPanel /> | Ensures UX resilience by displaying alternate content when a component fails to load. |
Resolved Mesh Nodes | <Component /> (output of resolveMesh ) | These are the dynamic UI fragments actually rendered into the DOM. |
- Route Handler: A user action (e.g., route change, tab switch) triggers the route handler, which determines the high-level layout context.
- DIM Mesh Resolver: This resolver fetches configuration or uses policy rules to identify which fragments or components to render.
- View Shell: This is the persistent layout structure (e.g., header, sidebar, footer) that defines named regions. These regions are placeholders for mesh nodes.
- Resolved Mesh Nodes: These are the final rendered components—resolved and injected dynamically. Each node may come from a different team, repository, or micro frontend deployment.
By separating the application shell from its dynamic content, DIM allows teams to independently ship UI updates, test variations, and progressively enhance the user interface without rebuilding the entire app.
- A route or event triggers the DIM Mesh Resolver.
- DIM resolves view fragments from local or remote registries.
- Fragments are injected into regions of the View Shell.
- Each component is isolated and may come from different deployment sources.
Implementation Examples
DIM enables runtime resolution and composition of UI fragments across surfaces. Below are some core code examples that show how it works in practice, along with deeper explanations:
1. Registering Mesh Components
registerMeshComponent('CheckoutSummary', () => import('./CheckoutSummary'));
This registers a view fragment called CheckoutSummary
. The function passed in is lazily invoked at runtime, allowing the system to defer loading until needed. These fragments can be independently versioned and deployed.
2. Resolving Mesh Fragments
const Component = resolveMesh('CheckoutSummary');
return <Component />;
DIM fetches the component dynamically via the registry. It abstracts away implementation details, so consumers only worry about the interface contract.
3. View Shell Composition (React)
<MeshRegion name="main" fallback={<Loading />}/>
<MeshRegion name="aside" policy="admin-only" />
<MeshRegion />
defines a slot that can be dynamically filled by the DIM resolver. Policies like admin-only
control visibility. Fallbacks ensure graceful degradation when resolution fails.
4. View Shell Composition (Web Component)
1 class CheckoutRegion extends HTMLElement {
2 async connectedCallback() {
3 const userId = this.getAttribute('user-id');
4 const isAdmin = this.getAttribute('admin') === 'true';
5 const Component = await resolveMesh('CheckoutSummary', { userId, isAdmin });
6 this.innerHTML = '';
7 const el = document.createElement('div');
8 el.appendChild(Component());
9 this.appendChild(el);
10 }
11 }
12 customElements.define('checkout-region', CheckoutRegion);
Explanation:
- Line 1: Defines a new Web Component class.
- Line 2: Uses the
connectedCallback
lifecycle method to start dynamic resolution. - Lines 3–4: Pulls runtime context from HTML attributes.
- Line 5: Dynamically resolves a component from the mesh registry with context data.
- Lines 6–9: Renders the resolved content into the shadow DOM.
- Line 12: Registers the custom element so it can be used like
<checkout-region user-id="123" admin="true" />
.
This approach allows the DIM system to work with any rendering layer—not just React.
Additional Code Example: Context-Based Resolution
DIM supports resolving components based on active user or feature context.
const regionPolicy = {
user: { isAdmin: true },
feature: ['new-checkout']
};
<MeshRegion name="checkout" policy={regionPolicy} fallback={<LegacyCheckout />} />
This enables:
- Conditional rendering per user role or feature toggle.
- Smooth transitions between legacy and new experiences.
Error Handling & Fallbacks
DIM uses the fallback pattern to handle loading or resolution errors. Each mesh node can specify its own fallback UI or error boundary.
<MeshRegion name="main" fallback={<ErrorPanel message="Section failed to load." />} />
Testing Strategies
DIM views can be tested using snapshot or integration tests:
- Validate composition via config.
- Mock component resolution to assert layout structure.
it('renders fallback if component fails', () => {
render(<MeshRegion name="aside" fallback={<span>Unavailable</span>} />);
expect(screen.getByText('Unavailable')).toBeInTheDocument();
});
Real-World Case Studies
🏢 Zalando – Dynamic Page Assembly
Background: As Zalando’s product catalog expanded across diverse European markets, they faced increasing pressure to deliver region-specific experiences quickly. Traditional monolithic deployments slowed iteration, especially when launching localized features or running A/B tests.
Problem:
- Teams couldn’t safely customize layouts for regional campaigns without redeploying the entire app.
- Updating shared layouts meant coordinating across multiple frontend groups.
- Pages grew bloated with conditional rendering and country-specific overrides, complicating performance and maintainability.
DIM Approach: Zalando implemented a runtime composition system inspired by DIM principles. Pages were structured as schemas describing layout and mesh slots. UI fragments were versioned and published to a central registry. Runtime resolution ensured the correct layout and UI were rendered for the user’s locale, brand, and feature set.
Developer Experience: Teams at Zalando reported faster onboarding, clearer ownership of UI responsibilities, and fewer regressions in shared surfaces. QA teams could test fragment behavior in isolation, and marketing could push layout tweaks without frontend intervention.
References:
- Zalando Tech Blog: Building Scalable Frontend Platforms
Results:
- Faster page load through lazy fragment loading.
- Teams deployed UI fragments without touching the shell.
- Consistent integration via shared contracts.
🏢 Atlassian – Pluggable UI Extensions
Background: Atlassian products power highly extensible experiences where third-party plugins are first-class citizens. Jira, Confluence, and Bitbucket all allow UI injection via extensibility APIs.
Problem:
- Partner developers needed a way to inject dynamic UIs without tightly coupling to core code.
- Plugin updates often broke or conflicted with Atlassian’s internal layout system.
- Product teams couldn’t easily enforce permissions or UI patterns across extensions.
DIM Approach: DIM was adapted into a mesh framework where each plugin declares where its view should render. Atlassian introduced “extension points” mapped to mesh regions, backed by policy engines that ensure plugins only load in permitted contexts.
Developer Experience: Plugin developers could preview integrations in a mesh-aware dev sandbox. Runtime tools allowed them to inspect slot availability and test multiple render paths. Internally, Atlassian teams could rollout mesh layout changes independently from plugin logic.
References:
- Atlassian Developer Guide: Forge and UI Extensions
Results:
- Accelerated partner onboarding.
- Safer upgrades with encapsulated renderers.
- Built-in policies to resolve UI only for users with access rights.
DIM in Practice: Patterns and Anti-Patterns
✅ Recommended Patterns
- Define regions and slots declaratively in the app shell: Establishes clear ownership of layout zones and encourages structured composition that is resilient to updates.
- Load components lazily and conditionally: Enhances performance and reduces time-to-interactive by loading only what is needed, based on feature flags or device type.
- Use a central registry or resolver API to abstract wiring logic: Prevents duplication and encourages consistency across feature modules and deployments.
- Separate view contracts from component implementations: Ensures that components can be swapped or versioned independently without breaking shared dependencies.
🚫 Anti-Patterns to Avoid
- Hardcoding imports for dynamic views: Eliminates the benefit of runtime composability and increases the need for redeployment on every layout change.
- Coupling mesh logic to business state or context: Creates tight coupling and risks breaking mesh boundaries when business logic shifts.
- Letting individual mesh nodes mutate global layout: Violates encapsulation and can introduce unpredictable rendering behavior.
- Reusing the same region for unrelated responsibilities: Causes overlapping content, ambiguity in intent, and poor maintainability. Always separate responsibilities with distinct regions.
Tooling and Dev Experience
- Mesh Resolver Tools: Developer overlays and visual debuggers let teams inspect which fragments are loaded, their sources, and their mount status at runtime.
- Storybook Mesh Testing: Enable isolated rendering and simulation of mesh regions with mocks, allowing UI fragments to be tested outside the main app shell.
- Runtime Toggle Managers: Integrate with feature flagging systems (e.g., LaunchDarkly, Unleash) to control mesh fragment inclusion based on A/B experiments or user roles.
- Registry Browsers: Provide real-time dashboards showing registered components, their status, and dependency mappings. Useful for debugging resolution failures or version mismatches.
DIM Benefits
DIM unlocks architectural advantages for modern, multi-platform frontend ecosystems:
- Portability: Fragments can be deployed independently and consumed across shells.
- Flexibility: Layouts adapt to device, permissions, or user roles without requiring new builds.
- Isolation: Teams can ship UI updates without stepping on each other's work.
- Testability: View modules can be mocked or tested independently.
- Extensibility: New views or plugins can be composed into existing shells using clear contracts.
Benefits of DIM
Benefit | Description |
---|---|
Runtime Composition | Dynamically load and inject components based on runtime context. |
Faster Iteration | Teams can update and deploy fragments without touching the main layout. |
Team Autonomy | Each mesh node can be independently built, owned, and tested by different teams. |
Device & Context Awareness | Components are selected based on feature flags, user roles, or platform types. |
Better Fault Isolation | Faulty nodes don’t break the entire UI—they can fail independently with fallbacks. |
Scalable Development Pattern | Encourages modular frontend design across distributed organizations. |
How DIM Fits with CEL and ECN
DIM does not work in isolation—it is part of a composable ecosystem:
- CEL (Composable Execution Layer) handles business logic and reusable workflows. It defines what happens.
- DIM (Dynamic Interface Mesh) controls where and how the UI is composed.
- ECN (Event-Driven Component Network) coordinates cross-component communication through an event bus.
Together, these pillars form a scalable, composable frontend system:
- CEL triggers behaviors
- DIM renders appropriate views
- ECN connects distributed components
Getting Started with DIM
Here’s a checklist to help you adopt DIM in your app:
✅ DIM Starter Checklist (Formatted)
Step | Task | Description |
---|---|---|
1 | Define shell layout | Declare named regions like main , aside , and footer using MeshRegion . |
2 | Set up component registry | Create a central resolver that supports registration, resolution, and versioning of fragments. |
3 | Create mesh-compatible components | Build UI fragments that are self-contained and deployable independently. |
4 | Configure resolution rules | Define rendering policies (e.g., by role, device, or feature flag). |
5 | Add fallbacks | Ensure every region has a loading or error fallback for graceful degradation. |
6 | Test in isolation | Validate fragment behavior using Storybook, mocks, or integration tests. |
DIM as a Mental Model
Think of DIM like DNS for UI: instead of resolving a domain to an IP address, DIM resolves view names to renderable components at runtime. This analogy helps developers visualize the decoupling between what gets rendered and how it's decided.
Testing View Contracts and Registry
To ensure consistent runtime resolution and access control, it’s essential to test both:
// Verifies view registry configuration
expect(meshRegistry.get('user-profile').policy).toContain('authenticated');
Use contract-based testing to validate what each mesh node expects and what it returns.
Deployment Considerations
- Registry CDN: Publish fragments to a CDN where the mesh resolver can access them based on routing or policies.
- Versioning Strategy: Use semantic versioning for mesh nodes so upgrades are controlled and predictable.
- Integrity Validation: Sign registry entries or enforce schema validation for secure composition.
- Offline Support: Mesh nodes should gracefully degrade when the registry or network is unavailable.
Summary
DIM introduces a powerful architectural shift: moving from static, tightly-coupled layout trees to runtime-resolved, policy-driven UI composition. It supports scalable frontend teams by allowing them to build and deploy UI fragments independently—while still contributing to a unified interface.
When paired with CEL and ECN, DIM becomes part of a distributed system for frontend logic, communication, and rendering—empowering teams to build faster, more resilient UIs at scale.