Adaptive Presentation Core (APC)
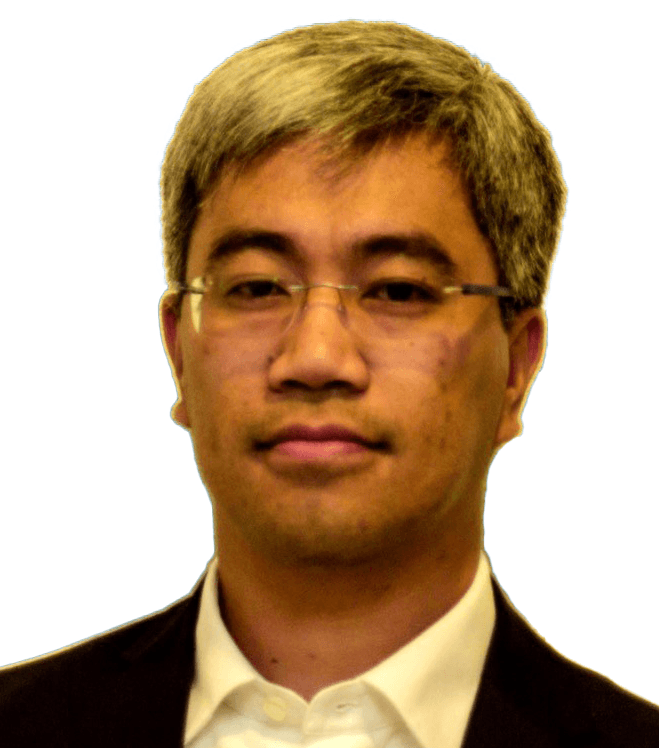
- Published on
Introduction
In a modern composable frontend, the user interface must adapt across a wide range of surfaces—desktop, mobile, embedded displays, and accessibility layers. The Adaptive Presentation Core (APC) serves as the responsive foundation of the system: a context-aware rendering layer that adapts layout, styling, interaction, and accessibility dynamically.
APC abstracts the logic that determines how something is rendered—based on where and for whom it's rendered. While CEL controls what happens, and DIM decides where content is composed, APC ensures content is presented optimally to the user.
Why We Need APC
As UIs scale across form factors, environments, and personalization contexts, rigid CSS and static component logic break down. APC addresses this by enabling responsive, intelligent, and adaptive presentation across a wide spectrum of devices, environments, and user needs. Below is a deeper explanation of the core motivations:
Responsive Rendering Logic: Traditional responsive design relies on brittle media queries. APC enables components to query context such as screen width, input method, or orientation and adapt their structure and behavior in real-time.
Contextual Styling: Rather than static CSS classes, APC propagates styling decisions via contextual data (e.g., dark mode, density preference). Components react to these settings dynamically, providing a more cohesive UX.
Accessibility and Assistive Support: APC integrates accessibility preferences like reduced motion, high contrast, or screen reader support directly into the render logic, reducing the need for external overrides or duplicated logic.
Surface-Aware Customization: A component might look and behave differently on a smartwatch, car infotainment system, or desktop browser. APC provides hooks to adjust rendering density, visual complexity, and animations accordingly.
Composition-Driven Presentation: For instance, a product grid might render in columns on desktop, become a swipeable list on mobile, or collapse into a dropdown on an embedded display. APC orchestrates these transitions without duplicating logic or layout configuration.
📘 Reference: Google’s Material Design Guidelines emphasize adaptive UIs across surfaces—APC codifies these in a componentized, composable architecture.
Historical Context and Prior Art
APC's design draws on several decades of frontend evolution. To appreciate its role, it helps to examine the shortcomings and breakthroughs in prior solutions.
Media Queries and Breakpoints
Traditional CSS media queries were a breakthrough for early responsive design. They allowed developers to create different layouts for screens of varying sizes. However, they are static, global, and lack component encapsulation—making them difficult to scale in large applications. In complex UI systems, breakpoints often become inconsistent and hard to manage across component libraries.
Tailwind and Utility-First CSS
Tailwind introduced a highly flexible, utility-driven way to style components with composable atomic classes. While Tailwind improves maintainability and scales well for design consistency, it operates largely at build time. It lacks awareness of runtime contexts like user input preferences (e.g. motion), accessibility settings, or environment (e.g. embedded app vs. kiosk).
Theme Providers and Design Tokens
Design systems often rely on theme providers (like styled-components’ ThemeProvider or CSS variables) and design tokens to support brand consistency and dark/light theming. These are effective for brand coherence but not for runtime adaptability. They typically lack fine-grained control based on input devices, screen density, or user accessibility requirements.
React Context and Render Props
React Context and render props provided powerful ways to inject and share state (like themes or device settings) across components. However, these tools require manual wiring, which often becomes verbose and difficult to scale without a unified system. APC leverages these underlying technologies but wraps them in consistent adaptive primitives and declarative rendering strategies that scale across environments.
📘 Related: React Aria by Adobe Spectrum leverages many APC-like principles to dynamically render adaptive UIs for accessibility and device support.
Architecture Overview
The APC architecture enables adaptive UIs by organizing rendering logic into layers that respond to platform signals, accessibility needs, and device capabilities. Rather than rely on brittle media queries or static themes, APC lets the UI tree react to context changes at runtime in a composable, testable way.
It revolves around three conceptual pillars:
Context Providers: Inject structured data like current surface type (e.g. mobile, desktop, embedded), preferred theme, motion preference, and color contrast mode into the component tree. This data flows through React Context (or similar system).
Adaptive Components: These are reusable presentational units that consume context to adjust layout, spacing, transitions, and child composition. They may delegate rendering based on template strategy (e.g., mobileStack vs. desktopGrid).
Render Strategies: These define how components adapt: whether by switching between layout variants, applying reduced motion, or changing DOM structure (e.g. dropdown vs. sidebar).
The following diagram shows the core architectural flow:
+----------------------------+
| View Context |
| (surface, theme, motion) |
+----------------------------+
↓
+----------------------------+
| Adaptive Presentation |
| - Layout Templates |
| - Component Adapters |
+----------------------------+
↓
+----------------------------+
| Platform UI Shell |
+----------------------------+
Implementation Examples
To implement APC effectively, developers build a combination of reusable context providers and adaptive components that listen to those contexts. Below are several examples using TypeScript, React, and Web Components.
TypeScript (Context + Hook)
This code defines a PresentationContext
that tracks rendering parameters across the app:
interface PresentationContext {
surface: 'mobile' | 'desktop' | 'tv';
theme: 'light' | 'dark';
motion: boolean;
}
const PresentationContext = createContext<PresentationContext>({
surface: 'desktop',
theme: 'light',
motion: true,
});
export const usePresentation = () => useContext(PresentationContext);
Explanation:
- interface PresentationContext: Defines a strongly typed context interface for UI presentation.
- createContext: Initializes a context with default rendering behavior (e.g., desktop surface).
- usePresentation: Custom hook to consume presentation data in any React component.
React Usage
const { surface, theme } = usePresentation();
return (
<Card layout={surface === 'mobile' ? 'stacked' : 'side-by-side'} theme={theme} />
);
Explanation:
- usePresentation(): Accesses the context to adapt layout and theme props.
- layout + theme props: Drive visual adaptation for device type and color scheme.
Web Component (with Context Attributes)
class AdaptiveCard extends HTMLElement {
connectedCallback() {
const surface = this.getAttribute('surface') || 'desktop';
this.innerHTML = surface === 'mobile'
? `<div class="stacked">Mobile Layout</div>`
: `<div class="side-by-side">Desktop Layout</div>`;
}
}
customElements.define('adaptive-card', AdaptiveCard);
Explanation:
- getAttribute('surface'): Reads the platform context directly from HTML.
- Conditional rendering: Renders the appropriate structure for the current surface.
- Web component API: Makes APC-compatible UIs usable in non-React environments.
Error Handling and Fallback Strategies
APC must fail gracefully when context is unavailable. Components should default to base layout:
const layout = context?.surface ?? 'desktop';
And provide reduced-motion alternatives or alt text for inaccessible states.
Real-World Case Studies
Case studies help contextualize how APC principles have been applied at scale across real-world products. These stories showcase how adaptive presentation improves performance, accessibility, and brand consistency across environments.
🏢 Adobe – Contextual UI with Spectrum
Problem: Adobe's diverse product suite—including Creative Cloud, Photoshop for iPad, and web-based tools like Express—required a unified UI library that could adapt seamlessly across desktop, mobile, and web platforms. Developers faced challenges in maintaining consistency and accessibility across these varied environments.
Solution: Adobe developed React Spectrum, a collection of libraries and tools designed to build adaptive, accessible, and robust user experiences. React Spectrum components are designed to work with mouse, touch, and keyboard interactions, built with responsive design principles to deliver a great experience across devices. (Adobe blog)
Results:
- Unified brand across surfaces
- Easier accessibility compliance
- Reusable layout components that adapt at runtime
🏢 Shopify – Responsive Checkout Engine
Problem: Shopify's checkout experience needed to adapt seamlessly to various contexts, including desktop, mobile, embedded POS, and headless commerce environments. Developers struggled with the limitations of the previous checkout customization methods, which were often brittle and required continuous upkeep.
Solution: Shopify introduced Checkout Extensibility, a new way of customizing the Shopify Checkout that's app-based, upgrade-safe, and more performant. This allowed developers to build adaptive cores with presentation strategies tailored for each surface and context. (Shopify Partners blog)
Results:
- Faster checkout iterations
- Reduced breakage in edge-case devices
- Shared component logic across all checkout views
APC in Practice: Patterns and Anti-Patterns
To effectively implement APC, teams should follow adaptive design best practices and avoid common pitfalls.
✅ Recommended Patterns
- Use context to propagate platform-level UI states: Centralize platform and accessibility preferences using providers like React Context. This enables composability and testability.
- Abstract layout rules into adaptive components: Avoid duplication by consolidating breakpoint logic and UI variants into a small set of adaptive component patterns.
- Use tokens, spacing, and theming based on context: Drive visual consistency and accessibility compliance by dynamically sourcing from theme tokens and density rules.
🚫 Anti-Patterns to Avoid
- Inline media queries inside components: Mixing CSS breakpoints into JSX makes logic brittle and hard to reuse.
- Hardcoded CSS values: Avoid fixed dimensions or spacing values that ignore surface or density preferences.
- Mixing content structure with presentation: Keep semantics (e.g., headings, sections) separate from visual adaptations like padding or flex layout. Use adapters or slots when layout varies. inside components
- Hardcoded CSS values
- Mixing content structure with presentation
Tooling and Developer Experience
Developer workflows benefit greatly from tooling that allows previewing and validating adaptive behavior in development:
- APC Playground: A multi-surface testing environment that renders UIs across mobile, tablet, desktop, and embedded shells.
- Preset Bundles: Predefined combinations of surface types, themes, and motion settings used for rapid testing or demos.
- Live Preview Switcher: An in-app toolbar or IDE plugin to toggle between context states (e.g., light/dark mode, reduced motion).
- Accessibility Simulators: Browser extensions or integrated simulators to audit and simulate screen readers, keyboard navigation, and color contrast requirements.
🛠 Reference Tools: Storybook Addons for Accessibility and Responsive Preview extend preview capabilities for APC implementations.
Benefits of APC
Benefit | Description |
---|---|
Contextual Presentation | Ensures each UI adapts appropriately to screen size and interaction method. |
Accessibility by Default | Forces teams to consider contrast, motion, and navigation across all UIs. |
Reuse Across Surfaces | One logic base, many presentation outputs. |
Designer/Developer Harmony | Codifies design intentions in reusable patterns. |
Systematic Theming | Unifies themes and variants across layout strategies. |
Getting Started with APC
Step | Task | Description |
---|---|---|
1 | Define platform UI context | Start with theme, motion, and surface properties. |
2 | Create context-aware components | Build components that adapt to changes in context. |
3 | Enable fallback behaviors | Ensure base rendering for unknown or degraded environments. |
4 | Integrate into layout and shell | Pass context via providers throughout layout tree. |
5 | Test across surfaces and features | Use simulators or preset environments to validate adaptive logic. |
Summary
APC completes the composable frontend triad by delivering an adaptable presentation layer for a multi-device, multi-user world. While CEL executes logic and DIM assembles views, APC renders each view in the most context-aware, user-friendly way possible.
This ensures not just composability—but accessibility, responsiveness, and platform harmony—are baked into the experience.
→ Next: How the Event-Driven Component Network (ECN) binds it all together.