Universal Interaction Framework (UIF)
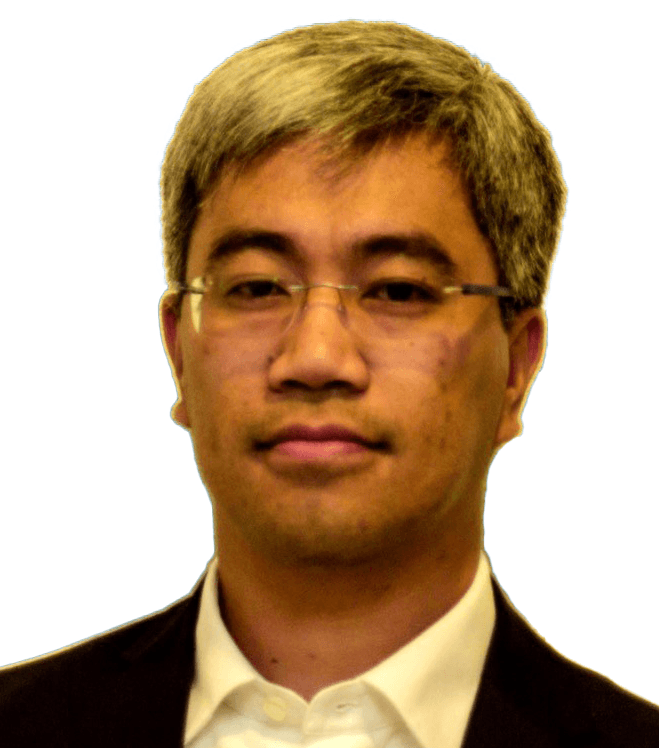
- Published on
A Platform-Agnostic Layer for Seamless User Experience
Introduction to UIF
The Universal Interaction Framework (UIF) is a composable, platform-agnostic interaction layer designed to unify the user experience across multiple runtimes, devices, and render strategies. It abstracts the details of how and where an interface is rendered—be it client-side, server-side, native, or headless—and allows developers to define interaction logic once and deploy it universally.
UIF decouples interaction behavior from rendering specifics, enabling teams to support multiple interfaces (e.g., web, mobile, embedded, kiosk) while maintaining a single interaction model and behavioral contract.
Why UIF?
Modern digital experiences are expected to be omnipresent—available on every device, in every format, and consistent in behavior. But creating interaction models that work across these mediums often leads to duplicated logic, platform-specific implementations, and maintenance nightmares.
UIF solves this by offering a framework where interaction logic—validation, state transitions, command handling, accessibility behaviors—is authored once, and then plugged into different presentation layers. This ensures cohesive interaction behavior without sacrificing the unique requirements of each platform.
Core Principles of UIF
Platform-Agnostic Interactions:
Define your interaction logic independently of how it’s rendered—no assumptions about DOM, native widgets, or markup.Declarative Interaction Contracts:
Interactions are described in declarative form, often as state machines or rule-driven handlers.Composable and Extendable:
UIF components are composable and pluggable. You can extend or override interaction logic for specific targets without rewriting the whole model.Adaptive Rendering Targets:
Whether rendered on the client, server, or precompiled to static assets, UIF enables uniform behavior.Accessibility and Behavior Parity:
Because behavior is centralized, accessibility and usability improvements apply globally.
How UIF Works
At its core, UIF is composed of three layers:
- Interaction Models: Define the state, transitions, and rules.
- Render Adapters: Render the interaction to a specific target (e.g., HTML DOM, React Native, Canvas).
- Interaction Context: Provides runtime state and behavioral orchestration.
Code Example: Button with Declarative Interaction
1. Define Interaction Model
// uif/models/buttonMachine.js
import { createMachine } from 'xstate';
export const buttonMachine = createMachine({
id: 'button',
initial: 'idle',
states: {
idle: {
on: {
HOVER: 'hovered',
PRESS: 'pressed'
}
},
hovered: {
on: {
LEAVE: 'idle',
PRESS: 'pressed'
}
},
pressed: {
on: {
RELEASE: 'idle'
}
}
}
});
This defines a universal interaction contract for a button that responds to hover, press, and release events.
2. Interaction Context Provider
// uif/context/InteractionProvider.js
import React, { createContext, useContext } from 'react';
import { useMachine } from '@xstate/react';
const InteractionContext = createContext();
export const InteractionProvider = ({ machine, children }) => {
const [state, send] = useMachine(machine);
return (
<InteractionContext.Provider value={{ state, send }}>
{children}
</InteractionContext.Provider>
);
};
export const useInteraction = () => useContext(InteractionContext);
This provides an interaction context to any render target—whether it’s React DOM, React Native, or WebGL.
3. Render Adapter for Web
// uif/adapters/ButtonWeb.js
import React from 'react';
import { useInteraction } from '../context/InteractionProvider';
const ButtonWeb = () => {
const { state, send } = useInteraction();
return (
<button
onMouseEnter={() => send('HOVER')}
onMouseLeave={() => send('LEAVE')}
onMouseDown={() => send('PRESS')}
onMouseUp={() => send('RELEASE')}
className={`btn ${state.matches('hovered') ? 'btn-hover' : ''} ${
state.matches('pressed') ? 'btn-active' : ''
}`}
>
Click Me
</button>
);
};
export default ButtonWeb;
Here, we’re mapping interaction events to UI events in the browser, while keeping interaction behavior decoupled.
UIF in Other Runtimes
React Native Adapter
// uif/adapters/ButtonNative.js
import React from 'react';
import { TouchableOpacity, Text } from 'react-native';
import { useInteraction } from '../context/InteractionProvider';
const ButtonNative = () => {
const { state, send } = useInteraction();
return (
<TouchableOpacity
onPressIn={() => send('PRESS')}
onPressOut={() => send('RELEASE')}
style={{
backgroundColor: state.matches('pressed') ? '#333' : '#555',
padding: 10,
borderRadius: 6
}}
>
<Text style={{ color: '#fff' }}>Click Me</Text>
</TouchableOpacity>
);
};
export default ButtonNative;
With this adapter, you get the same behavior, just expressed in the native runtime.
Benefits of UIF
Code Reuse Across Platforms
Define once, use everywhere. Interaction behavior is authored once and deployed across platforms.Consistency in Behavior and UX
Users get a consistent experience across web, mobile, and embedded systems.Faster Feature Rollouts
When interaction behavior is centralized, you can introduce new behaviors or fixes without touching every front end.Improved Accessibility
Accessibility behavior can be injected once and shared globally—reducing the risk of regressions.Easier QA and Testing
Interaction logic can be unit tested independently of the UI rendering engine.
Use Cases
Design Systems
UIF can power design systems that span React, React Native, and Web Components with a unified interaction layer.Headless CMS Frontends
Deliver a frontend that works both server-rendered and client-rendered with identical behavioral rules.IoT and Kiosk Interfaces
Use UIF to power buttons, screens, and sensors with shared logic across embedded and web UIs.
Conclusion
The Universal Interaction Framework (UIF) redefines how we build frontend interactions across platforms. By abstracting interaction from presentation, UIF offers a clean separation of concerns, enabling developers to build platform-agnostic UIs that maintain a unified behavioral model.
UIF is the foundation for teams who want to build once and run everywhere, while preserving flexibility, performance, and maintainability. It pairs well with Composable Execution Layer (CEL), Adaptive Presentation Core (APC), and Event-Driven Component Network (EDCN) as part of a fully composable frontend architecture.
Let me know if you'd like this turned into a PDF, published format, or visual diagrams to pair with it!