Event-Driven Component Network (EDCN)
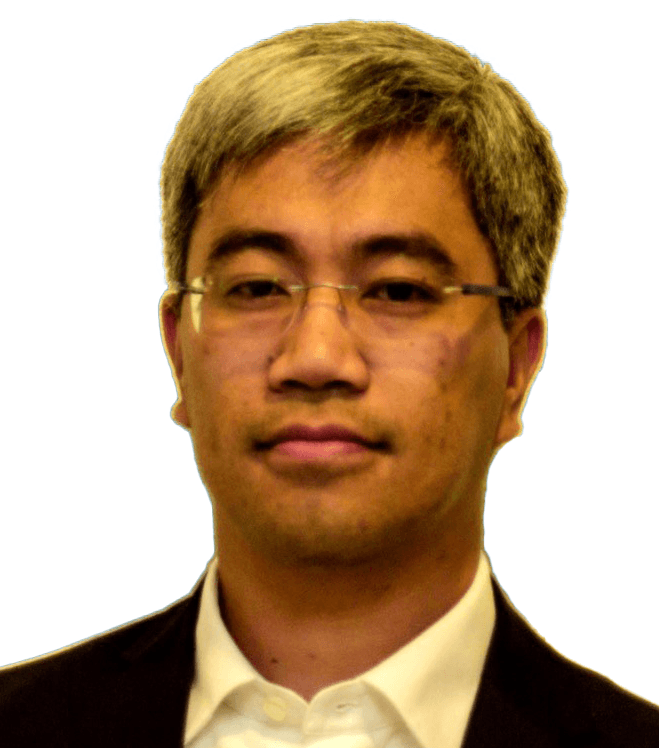
- Published on
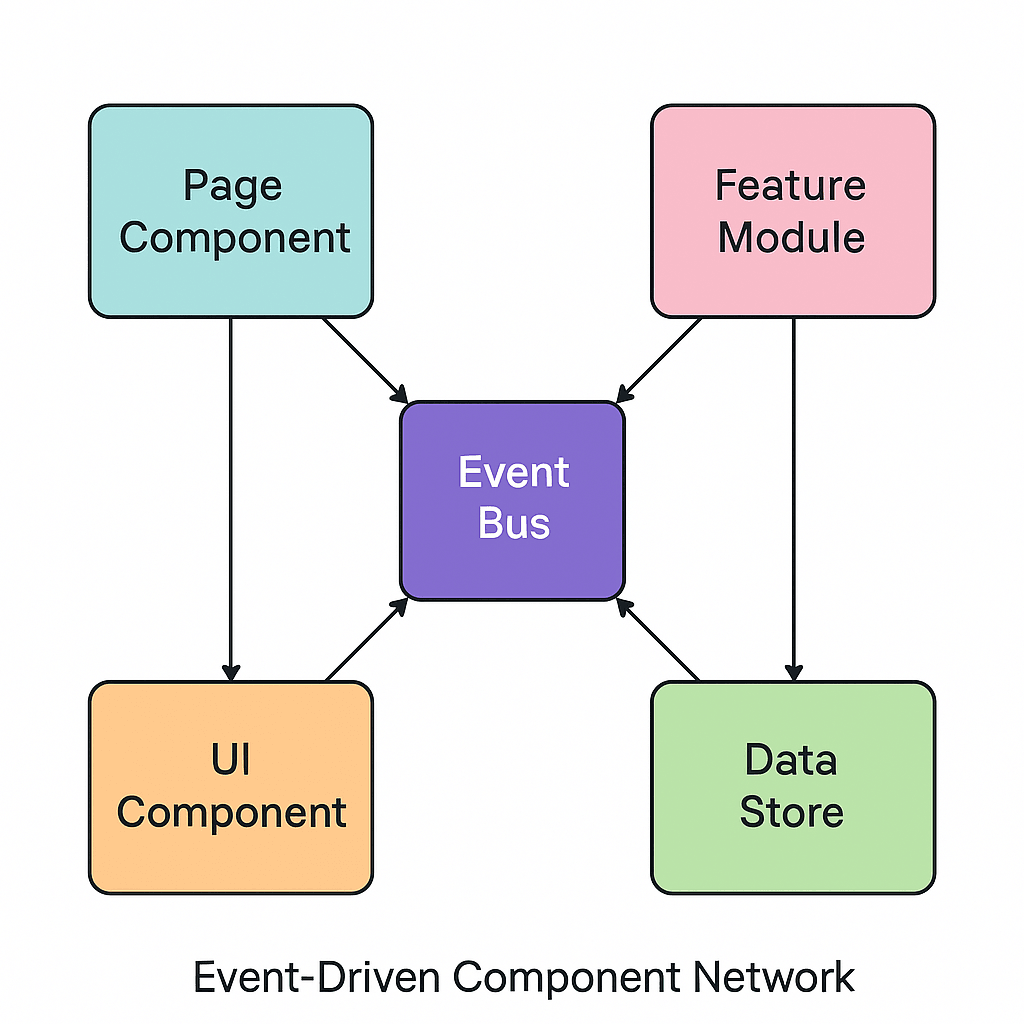
A Scalable, Responsive Architecture for Dynamic Systems
Introduction to EDCN
The Event-Driven Component Network (EDCN) is a next-generation architectural pattern designed to facilitate scalable, responsive, and highly dynamic systems. Built around the core principle of event-driven design, EDCN enables systems to handle asynchronous events in a robust and modular way. It allows components in a system to communicate and respond to events without direct dependencies, promoting decoupling and flexibility.
EDCN is ideal for building complex, real-time applications that need to scale easily and handle high levels of concurrency, such as in IoT, real-time analytics, microservices, and distributed systems.
Why EDCN?
As systems grow in complexity and scale, traditional request-response models often struggle to maintain responsiveness and scalability, especially under high concurrency or asynchronous workloads. EDCN addresses this challenge by shifting the focus to events as the main driver of communication between components.
By decoupling components and enabling them to react to events asynchronously, EDCN allows systems to scale horizontally, handle real-time data more efficiently, and remain responsive under load. This makes it particularly effective for distributed systems and applications that require high levels of scalability, fault tolerance, and real-time interaction.
Core Principles of EDCN
Event-Driven Architecture: EDCN relies on the publish-subscribe model, where components are event producers (publishers) and event consumers (subscribers). This architecture allows components to communicate asynchronously without tightly coupling them, improving flexibility and scalability.
Decoupled Components: Components in an EDCN system are designed to be loosely coupled. They don’t directly depend on one another but instead react to events. This decoupling reduces the risk of cascading failures and makes it easier to maintain and scale the system.
Asynchronous Communication: Events are delivered asynchronously to components, allowing for non-blocking communication. This enhances system responsiveness and ensures that components can continue processing while waiting for events to be handled.
Real-Time Interaction: EDCN is particularly effective in systems that need to respond to real-time events, such as user input, sensor data, or external triggers. It ensures that components react quickly and efficiently to changes in the environment.
How EDCN Works: Architecture Breakdown
The EDCN architecture is made up of several key components that work together to enable asynchronous, event-driven communication. These include Event Producers, Event Consumers, Event Brokers, and Event Handlers.
1. Event Producers
Event producers are components that generate events based on internal state changes or external triggers. These events can be anything from user actions, system states, or external data sources (e.g., APIs, sensors, etc.).
Example: Event Producer in React
// ButtonClickProducer.js
import React from 'react';
const ButtonClickProducer = ({ onClick }) => {
const handleClick = () => {
const event = { type: 'button-clicked', payload: { timestamp: Date.now() } };
onClick(event); // Send the event to the event broker or handler
};
return <button onClick={handleClick}>Click Me</button>;
};
export default ButtonClickProducer;
In this example, the ButtonClickProducer component generates an event each time the button is clicked. The event is passed to the Event Broker or Event Handler for further processing.
2. Event Consumers
Event consumers are components that listen for specific events and act accordingly. Consumers subscribe to particular event types and execute appropriate logic when the event occurs.
Example: Event Consumer in React
// EventConsumer.js
import React, { useState, useEffect } from 'react';
const EventConsumer = ({ event }) => {
const [message, setMessage] = useState('');
useEffect(() => {
if (event && event.type === 'button-clicked') {
setMessage(`Button clicked at: ${new Date(event.payload.timestamp).toLocaleTimeString()}`);
}
}, [event]);
return <div>{message}</div>;
};
export default EventConsumer;
The EventConsumer component listens for events such as 'button-clicked'
and updates the UI based on the event data.
3. Event Brokers
The Event Broker is responsible for managing the flow of events between producers and consumers. It acts as a central hub where events are published by producers and subscribed to by consumers. The event broker is typically implemented using an event bus or messaging queue.
Example: Event Broker Implementation (Simple)
// EventBroker.js
class EventBroker {
constructor() {
this.subscribers = {};
}
subscribe(eventType, callback) {
if (!this.subscribers[eventType]) {
this.subscribers[eventType] = [];
}
this.subscribers[eventType].push(callback);
}
publish(event) {
const { type } = event;
if (this.subscribers[type]) {
this.subscribers[type].forEach(callback => callback(event));
}
}
}
export const eventBroker = new EventBroker();
In this example, the EventBroker class provides methods for subscribing to and publishing events. The subscribe
method allows consumers to listen for specific event types, and the publish
method triggers all the subscribers to act on the event.
4. Event Handlers
Event handlers are the components that process the event once it’s consumed by the system. These handlers may perform complex logic, update the state of the system, or trigger other actions based on the event.
Example: Event Handler in React
// EventHandler.js
import { useEffect } from 'react';
import { eventBroker } from './EventBroker';
import ButtonClickProducer from './ButtonClickProducer';
import EventConsumer from './EventConsumer';
const EventHandler = () => {
useEffect(() => {
const handleButtonClick = (event) => {
console.log('Event received:', event);
// Handle the event (e.g., update state, perform logic)
};
eventBroker.subscribe('button-clicked', handleButtonClick);
return () => {
// Clean up subscription when component unmounts
eventBroker.unsubscribe('button-clicked', handleButtonClick);
};
}, []);
return (
<div>
<ButtonClickProducer onClick={(event) => eventBroker.publish(event)} />
<EventConsumer event={{ type: 'button-clicked', payload: { timestamp: Date.now() } }} />
</div>
);
};
export default EventHandler;
Here, EventHandler subscribes to the button-clicked
event and processes it using a callback function.
Benefits of EDCN
Loose Coupling: EDCN promotes decoupling of components. Producers and consumers don’t need to know about each other directly, which enhances system flexibility and maintainability.
Scalability: The event-driven nature of EDCN makes it easy to scale components independently. You can add more consumers, producers, or brokers as needed without disrupting the system.
Real-Time Processing: EDCN is ideal for systems that need to handle real-time events, such as stock price updates, sensor data streams, or user interactions in highly dynamic environments.
Asynchronous Communication: Since events are handled asynchronously, the system remains responsive and can handle high loads without blocking the main execution thread.
Fault Tolerance: Components in an event-driven system are loosely coupled, which means that failure in one component does not necessarily affect the others. Event brokers can ensure delivery even if a consumer is temporarily unavailable.
Applications of EDCN
1. Real-Time Systems
EDCN is ideal for real-time systems, such as financial trading platforms, gaming systems, or real-time analytics, where components need to react to events with minimal latency.
2. Microservices and Distributed Systems
In microservices architectures, EDCN enables decoupled communication between services, allowing for asynchronous messaging that is efficient and scalable.
3. IoT Systems
EDCN is well-suited for Internet of Things (IoT) systems, where devices generate events that need to be processed asynchronously. Each device can produce events that are consumed by appropriate handlers without direct dependency on other devices.
Conclusion
The Event-Driven Component Network (EDCN) provides a powerful framework for building scalable, responsive, and highly dynamic systems. By utilizing an event-driven architecture, EDCN enables components to operate asynchronously, decoupling them for better scalability and flexibility.
EDCN is perfect for systems that require real-time event processing, such as microservices, IoT systems, and distributed architectures. It improves system performance by allowing components to act on events without blocking and enhances fault tolerance by decoupling producers and consumers.