Adaptive Presentation Core (APC)
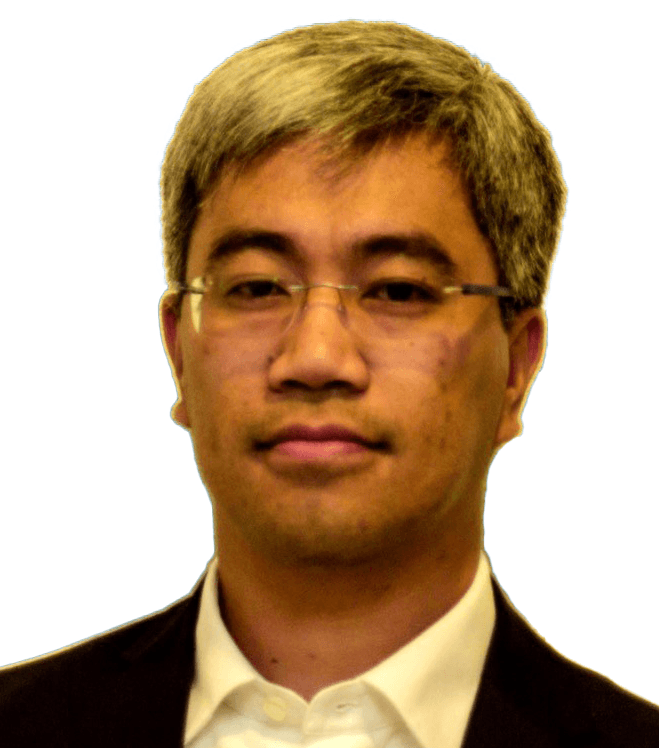
- Published on
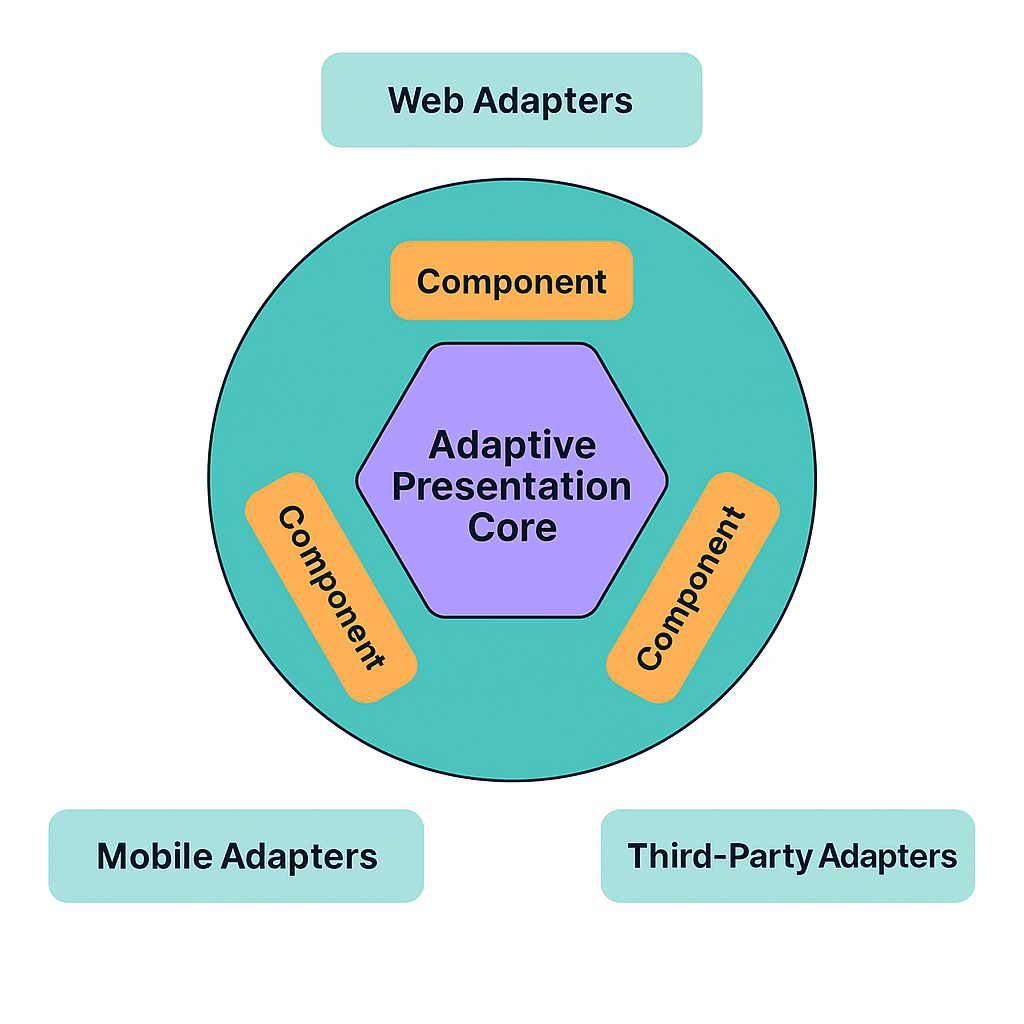
Empowering Scalable and Dynamic UIs
Introduction to APC
The Adaptive Presentation Core (APC) is an advanced architectural pattern designed to facilitate the dynamic adaptation of user interfaces based on the context, environment, and user behavior. It empowers developers to create scalable, context-aware, and responsive user interfaces that adapt in real-time to changing conditions. APC provides the foundation for building UIs that can adjust their layout, design, and behavior based on various factors such as device type, user preferences, and data state.
Why APC?
As applications become more complex and need to support a diverse range of platforms and user conditions, it is essential to rethink how UIs adapt to those variables. APC addresses this challenge by offering a flexible, data-driven, and contextual UI architecture. With APC, UIs are no longer static; they are adaptive, adjusting automatically based on the current context and user interactions.
APC enables developers to focus on creating user interfaces that dynamically respond to their environment, improving the user experience without requiring manual updates to each view.
Core Principles of APC
Context-Aware Adaptation: APC allows UIs to adapt based on contextual data, such as screen size, device orientation, user preferences, or environmental factors (e.g., network status). It ensures that the user interface always delivers an optimal experience regardless of the device or situation.
Component-Based Architecture: APC emphasizes the use of modular components that can be dynamically adapted to different contexts. Components are designed to be reusable and responsive, making it easier to maintain consistency across the UI.
Dynamic Rendering: With APC, the UI can be rendered dynamically, meaning that the components and layout adjust based on real-time data and user input. This approach supports both static and dynamic UI changes without requiring a complete page reload.
Seamless Integration with Existing Systems: APC integrates seamlessly with backend services, third-party libraries, and existing UI frameworks, ensuring that it can be adopted incrementally into existing systems. APC can interact with React, Vue, or Angular while maintaining a high level of flexibility.
How APC Works: Architecture Breakdown
The APC architecture consists of several core layers: Context Providers, Dynamic Components, Adaptive View Renderers, and State Managers. These layers work together to enable adaptive UI behavior that responds to user context and environmental conditions.
1. Context Providers
Context providers supply the necessary environmental information (such as device type, screen size, user preferences, etc.) that determines how the UI components should behave and adapt.
Example: Context Provider for Device Type
// DeviceContext.js
import React, { createContext, useState, useEffect } from 'react';
const DeviceContext = createContext();
const DeviceProvider = ({ children }) => {
const [deviceType, setDeviceType] = useState('desktop');
useEffect(() => {
const updateDeviceType = () => {
const width = window.innerWidth;
setDeviceType(width < 768 ? 'mobile' : 'desktop');
};
window.addEventListener('resize', updateDeviceType);
updateDeviceType();
return () => window.removeEventListener('resize', updateDeviceType);
}, []);
return (
<DeviceContext.Provider value={deviceType}>
{children}
</DeviceContext.Provider>
);
};
export { DeviceContext, DeviceProvider };
In this example, the DeviceProvider component listens for changes in the window's width and provides the current device type (mobile or desktop) to the components that need it. This context can be used to dynamically adjust UI components based on the device type.
2. Dynamic Components
Dynamic components in APC are modular, reusable, and capable of adapting their content or appearance based on the context provided by the context providers. These components are designed to be responsive and flexible, adjusting to both data changes and environmental factors.
Example: Adaptive Button Component in React
// AdaptiveButton.js
import React, { useContext } from 'react';
import { DeviceContext } from './DeviceContext';
const AdaptiveButton = ({ label, onClick }) => {
const deviceType = useContext(DeviceContext);
const buttonStyle = {
padding: deviceType === 'mobile' ? '10px 15px' : '15px 20px',
fontSize: deviceType === 'mobile' ? '14px' : '18px',
backgroundColor: deviceType === 'mobile' ? 'blue' : 'green',
color: 'white',
borderRadius: '5px',
};
return (
<button style={buttonStyle} onClick={onClick}>
{label}
</button>
);
};
export default AdaptiveButton;
Here, the AdaptiveButton component uses the device type from the DeviceContext to adjust its style and behavior dynamically. On mobile devices, the button will be smaller with different padding and background color compared to its desktop counterpart.
3. Adaptive View Renderers
The Adaptive View Renderers are responsible for dynamically assembling UI components based on the current context and user interaction. These renderers can change the composition of the UI based on state, data, or contextual information provided by the context providers.
Example: Adaptive User Profile Page in React
// AdaptiveUserProfile.js
import React, { useState, useEffect, useContext } from 'react';
import { DeviceContext } from './DeviceContext';
import AdaptiveButton from './AdaptiveButton';
const AdaptiveUserProfile = () => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
const deviceType = useContext(DeviceContext);
useEffect(() => {
// Simulate fetching user data
setTimeout(() => {
setUser({ name: 'John Doe', age: 30, email: 'john.doe@example.com' });
setLoading(false);
}, 2000);
}, []);
if (loading) {
return <div>Loading...</div>;
}
return (
<div style={{ padding: deviceType === 'mobile' ? '10px' : '20px' }}>
<h1>{user.name}</h1>
<p>Age: {user.age}</p>
<p>Email: {user.email}</p>
<AdaptiveButton label="Edit Profile" onClick={() => alert('Edit clicked')} />
</div>
);
};
export default AdaptiveUserProfile;
In the AdaptiveUserProfile component, we dynamically render the user profile page and use the AdaptiveButton to adjust the UI based on the device context. If the device is mobile, the padding and layout will adapt accordingly.
4. State Managers
State managers in APC handle the global application state and provide a central point for managing and synchronizing data across different parts of the UI. They ensure that changes in data or context are propagated throughout the application, triggering the necessary UI updates.
Example: Global State Management with React's useState
and useContext
// GlobalState.js
import React, { createContext, useState, useContext } from 'react';
const GlobalStateContext = createContext();
const GlobalStateProvider = ({ children }) => {
const [globalState, setGlobalState] = useState({ theme: 'light' });
return (
<GlobalStateContext.Provider value={{ globalState, setGlobalState }}>
{children}
</GlobalStateContext.Provider>
);
};
const useGlobalState = () => useContext(GlobalStateContext);
export { GlobalStateProvider, useGlobalState };
In this example, GlobalStateContext is used to manage the global state (in this case, the theme of the application). Components can use the useGlobalState
hook to access or update the global state.
Benefits of APC
Context-Aware UI: APC ensures that UIs adapt dynamically based on the current context. Whether it’s screen size, device type, or user preferences, APC allows the UI to adjust in real-time, improving user experience.
Modular and Reusable Components: The component-based architecture of APC ensures that components are reusable and can be dynamically adjusted to fit different contexts. This leads to cleaner code and easier maintenance.
Real-Time Adaptation: APC allows for real-time adaptation of the UI based on external factors (such as device changes, data updates, or user interactions), creating a seamless experience for the end user.
Scalability: APC’s modular design allows for easy scaling of the application. New components and contexts can be introduced without disrupting the existing system.
Applications of APC
1. Responsive UIs
APC is ideal for building responsive UIs that adapt to different devices, screen sizes, or orientations. It simplifies the process of handling multiple device layouts and ensuring that the application remains usable across various devices.
2. Data-Driven Interfaces
APC is well-suited for data-driven applications where the UI needs to update dynamically based on real-time data or user interactions. This includes use cases like dashboards, e-commerce platforms, and social media apps.
3. Multi-Platform Support
APC’s adaptability makes it perfect for applications that need to work across multiple platforms, such as web, mobile, and desktop. The same underlying logic can be reused across platforms with minor adjustments.
Conclusion
The Adaptive Presentation Core (APC) is a powerful architecture for building dynamic, scalable, and responsive UIs. It leverages context-aware adaptation, dynamic rendering, and modular components to create user interfaces that adjust seamlessly to different environments and user conditions.
By adopting APC, developers can build more flexible, responsive, and context-aware UIs that enhance the overall user experience. With APC, UIs no longer have to be static or rigid; they can adapt to the user’s needs in real-time.